What is State in React?
-
A state might hold the number of times a button is clicked.
-
When the button is clicked, the state changes and React updates the button's display to show the new count.
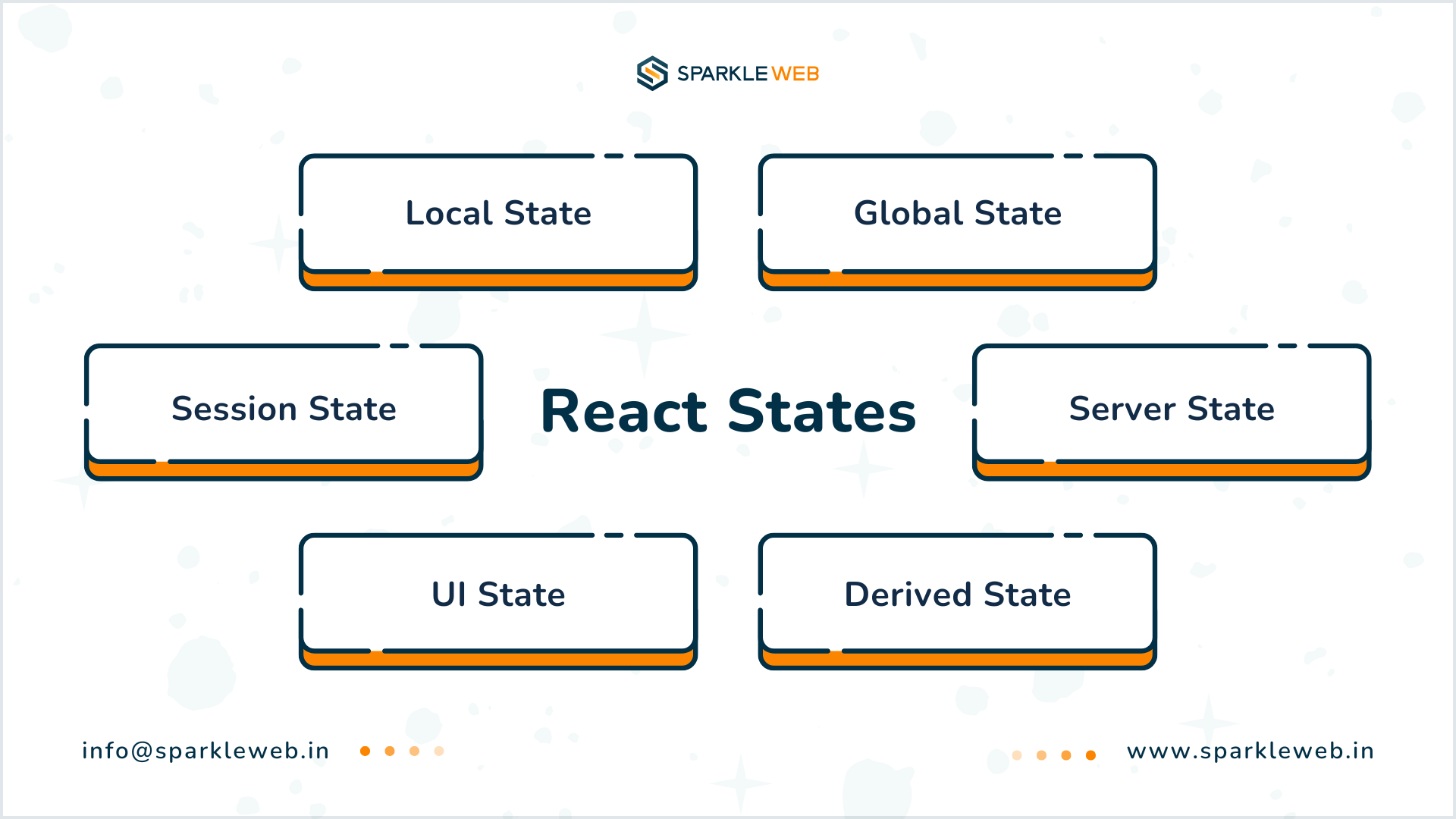
1. Local State
-
Managing form inputs (e.g., text fields, checkboxes).
-
Showing or hiding elements (like modals or tooltips).
- Switching between light and dark themes.
Here’s an example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0); // Initialize state with 0
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
-
useState is used to create a state variable called count.
-
setCount is the function used to update the state.
- When the button is clicked, setCount increases the value of count by 1.
-
Handling user input in forms.
-
Toggling the visibility of UI elements.
- Keeping track of simple component-specific values.
2. Global State
-
User login information (e.g., checking if the user is logged in).
-
App-wide themes (like dark mode or light mode).
- Data fetched from an API that needs to be accessible in multiple components.
-
Context API (a React feature).
-
Redux (a state management library).
- Zustand (a lightweight library).
import React, { createContext, useContext, useState } from 'react';
// Create a Context
const UserContext = createContext();
function App() {
const [user, setUser] = useState({ name: "John Doe" });
return (
<UserContext.Provider value={{ user, setUser }}>
<Profile />
</UserContext.Provider>
);
}
function Profile() {
const { user } = useContext(UserContext); // Access global state
return <p>Welcome, {user.name}!</p>;
}
-
The UserContext is used to share the user state across multiple components.
-
The Profile component can access and display the user data without passing it as a prop.
-
Managing user authentication (e.g., login/logout).
-
App-wide settings (e.g., themes, language).
- Sharing data between unrelated components.
3. Server State
-
Fetch data from a server.
-
Sync data between the front end and back end.
- Cache frequently used data.
-
React Query: Simplifies server state management.
-
Axios or Fetch API: Handles HTTP requests manually.
import { useQuery } from 'react-query';
function Users() {
const { data, isLoading, error } = useQuery('users', () =>
fetch('/api/users').then(res => res.json())
);
if (isLoading) return <p>Loading...</p>;
if (error) return <p>Error loading data</p>;
return (
<ul>
{data.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
- useQuery fetches data and handles loading or error states automatically.
-
Fetching user information, product listings, or posts from an API.
-
Keeping local data updated with server changes.
4. Derived State
function ShoppingCart({ items }) {
const total = items.reduce((sum, item) => sum + item.price, 0);
return <p>Total Price: ${total}</p>;
}
- The total value is calculated every time the items array changes.
-
Summarizing data (e.g., totals, averages).
-
Transforming or filtering data before display.
5. UI State
-
Showing or hiding modals, dropdowns, or tooltips.
-
Managing loading spinners or progress bars.
function Modal() {
const [isOpen, setIsOpen] = useState(false);
return (
<div>
<button onClick={() => setIsOpen(true)}>Open Modal</button>
{isOpen && (
<div className="modal">
<p>This is a modal</p>
<button onClick={() => setIsOpen(false)}>Close</button>
</div>
)}
</div>
);
}
-
Modals and popups.
-
Controlling dropdown menus.
- Displaying loading indicators.
6. Session State
-
Shopping carts.
-
Storing temporary preferences.
function useSessionStorage(key, initialValue) {
const [value, setValue] = useState(() => {
const saved = sessionStorage.getItem(key);
return saved ? JSON.parse(saved) : initialValue;
});
const save = (newValue) => {
setValue(newValue);
sessionStorage.setItem(key, JSON.stringify(newValue));
};
return [value, save];
}
Choosing the Right State
Sparkle Web Can Support
Contact us today to start your next project!
Vikas Mishra
A highly skilled Angular & React Js Developer. Committed to delivering efficient, high-quality solutions by simplifying complex projects with technical expertise and innovative thinking.
Reply