What is Angular 17?
What’s New in Angular 17?
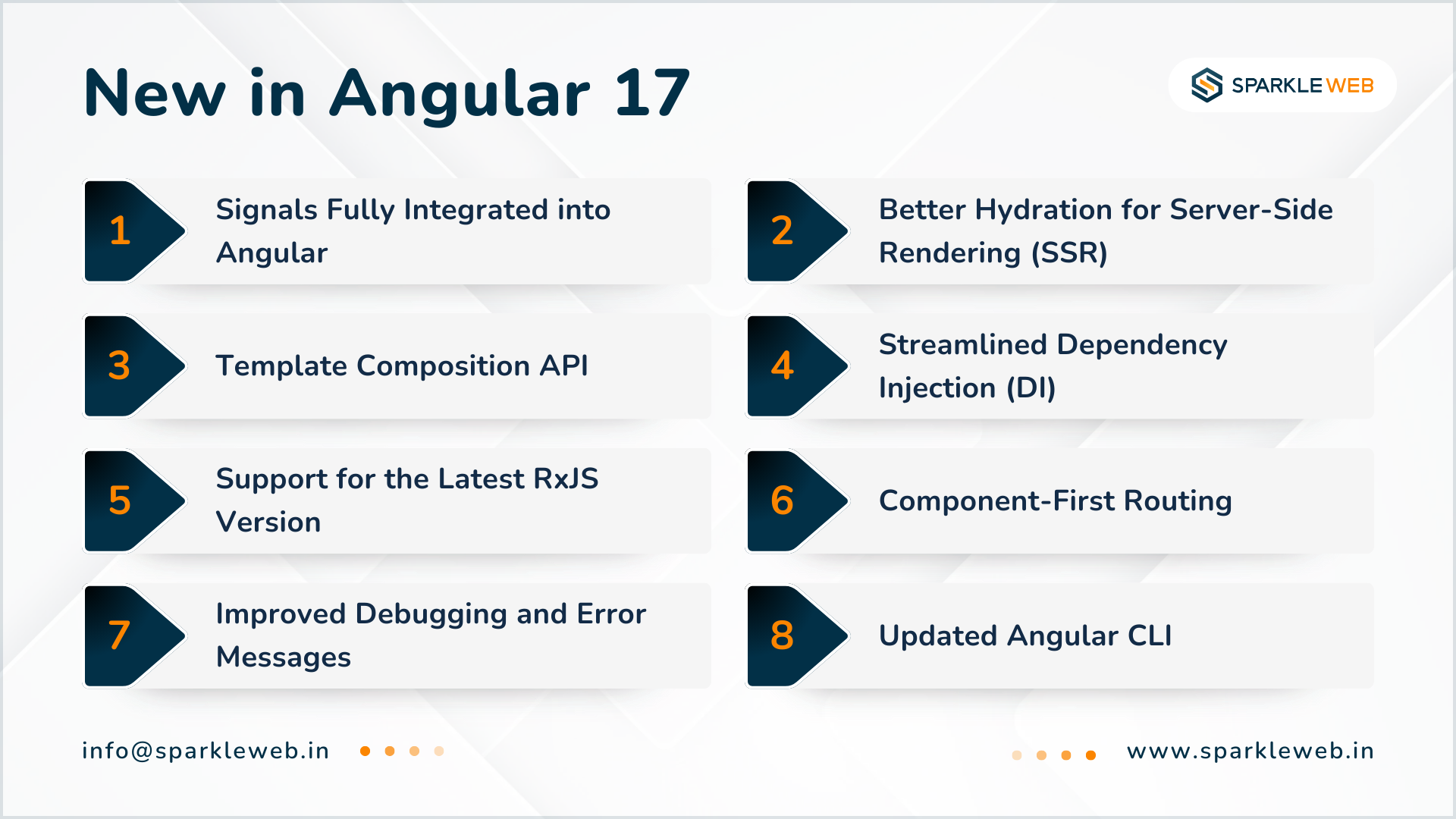
1. Signals Fully Integrated into Angular
Key Benefits of Signals
-
Fine-grained Reactivity: Signals track changes in your data and update only the affected components, making your app faster and more efficient.
-
Simplified State Management: Unlike traditional methods like two-way binding or RxJS, Signals make managing application data much easier.
- Easy Integration: Signals can be added to existing Angular applications without breaking anything.
Example
import { signal } from '@angular/core';
const counter = signal(0);
function increment() {
counter.set(counter() + 1);
}
Here, the signal is used to track the value of the counter. When you call the increment function, it updates the counter value, and Angular takes care of re-rendering any components that depend on it.
2. Better Hydration for Server-Side Rendering (SSR)
Benefits of Improved Hydration
-
Faster Load Times: Your app loads quicker because Angular minimizes the JavaScript needed for hydration.
-
SEO Optimization: Great for websites like blogs or e-commerce platforms where search engines need to index pages effectively.
- Seamless User Experience: The transition from static content (loaded by the server) to dynamic interactions (handled by the browser) is smoother.
Use Cases
-
E-commerce websites: For showcasing products and improving search engine rankings.
-
Content-heavy websites: Blogs or news portals benefit from faster loading and better interactivity.
3. Template Composition API
Benefits
-
Improved Readability: This makes the structure of your app clearer and easier to understand.
-
Reusable Templates: This avoids repeating the same code, saving time and reducing errors.
Example
@Component({
selector: 'app-header',
standalone: true,
template: '<h1>{{ title }}</h1>',
})
export class HeaderComponent {
title = 'Welcome to Angular 17!';
}
Here, the HeaderComponent is modular and can be reused across different parts of your app.
4. Streamlined Dependency Injection (DI)
What’s New in Angular 17’s DI?
-
Improved Performance: Faster app initialization by resolving dependencies more efficiently.
-
Tree-Shakable Services: Angular automatically removes unused services, making your app smaller and faster.
5. Support for the Latest RxJS Version
-
Smaller Bundle Sizes: Reduces the size of your app by removing unused code.
-
Better Compatibility with Signals: Works seamlessly with the new Signals feature.
- Improved Performance: Faster and more reliable reactive programming.
How to Upgrade RxJS
Simply use the following Angular CLI command:
ng update @angular/core @angular/cli
6. Component-First Routing
Benefits
-
Easier Configuration: Define routes directly in your components, reducing complexity.
-
Standalone Components: No need for extra modules, making your app more modular.
Example
const routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
];
7. Improved Debugging and Error Messages
Key Improvements
-
Clearer Error Messages: Provides step-by-step instructions to solve issues.
-
Better Stack Traces: This makes it easier to understand where the problem occurred.
8. Updated Angular CLI
New Features in the CLI
-
Support for Signals: Quickly add Signals and SSR templates to your project.
-
Faster Builds: Uses ESBuild to improve build speed and efficiency.
- New Commands: Simplifies common tasks like adding hydration or standalone components.
Why Upgrade to Angular 17?
How to Upgrade to Angular 17
Upgrading is simple. Just run the following command in your project:
ng update @angular/core @angular/cli
Work with Us
Contact us today to bring Angular 17’s new features to your next project and elevate your web application!
Vikas Mishra
A highly skilled Angular & React Js Developer. Committed to delivering efficient, high-quality solutions by simplifying complex projects with technical expertise and innovative thinking.
Reply