Why is a DICOM Viewer Important in a Hospital Management System?
-
View X-rays and other medical images directly inside the HMS.
-
Zoom in, pan, and adjust images for a clearer diagnosis.
- Store and retrieve images easily without needing external software.
Market Insights
What You Need Before You Start
-
cornerstone.js (to load and display images)
-
dicom-parser (to process DICOM files)
- OHIF Viewer (a pre-built DICOM viewer based on React)
Step-by-Step Guide to Integrate DICOM Viewer in React
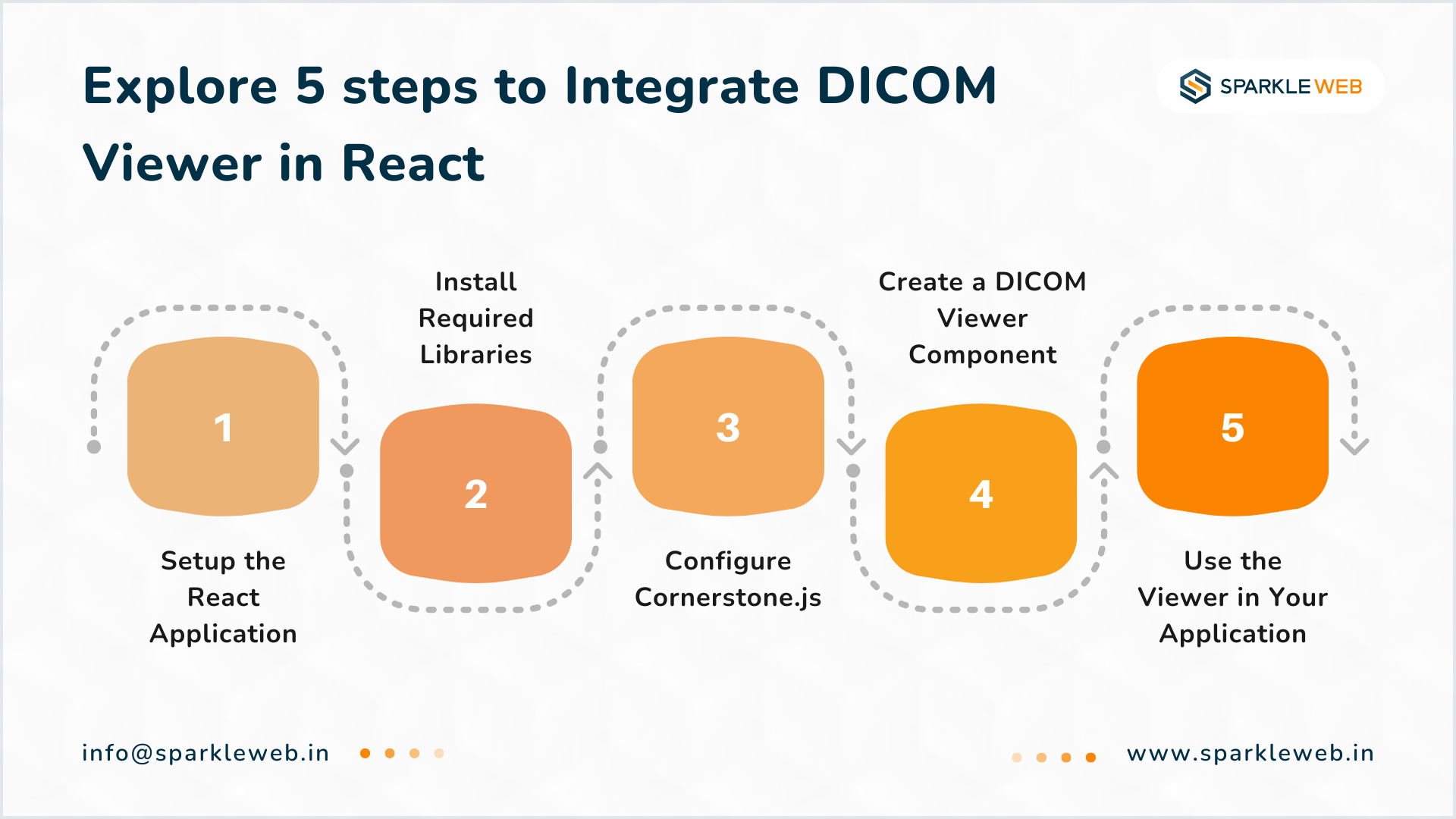
Step 1: Set Up a React Application
npx create-react-app dicom-viewer-app
cd dicom-viewer-app
npm start
Step 2: Install Required Libraries
npm install cornerstone-core cornerstone-tools cornerstone-wado-image-loader dicom-parser
Step 3: Configure Cornerstone.js
In your React project, set up cornerstone and cornerstoneWADOImageLoader to handle DICOM files.
import cornerstone from 'cornerstone-core';
import cornerstoneWADOImageLoader from 'cornerstone-wado-image-loader';
import dicomParser from 'dicom-parser';
cornerstoneWADOImageLoader.external.cornerstone = cornerstone;
cornerstoneWADOImageLoader.external.dicomParser = dicomParser;
export const initializeCornerstone = () => {
cornerstoneWADOImageLoader.configure({
beforeSend: function(xhr) {
xhr.setRequestHeader('Accept', 'application/dicom');
},
});
};
Step 4: Create a DICOM Viewer Component
import React, { useEffect, useRef } from 'react';
import cornerstone from 'cornerstone-core';
import cornerstoneWADOImageLoader from 'cornerstone-wado-image-loader';
const DicomViewer = ({ dicomUrl }) => {
const viewerRef = useRef(null);
useEffect(() => {
cornerstone.enable(viewerRef.current);
cornerstone.loadAndCacheImage(dicomUrl).then((image) => {
cornerstone.displayImage(viewerRef.current, image);
});
return () => cornerstone.disable(viewerRef.current);
}, [dicomUrl]);
return <div ref={viewerRef} style={{ width: '512px', height: '512px', backgroundColor: 'black' }} />;
};
export default DicomViewer;
Step 5: Use the DICOM Viewer Component in Your App
Now, import the DicomViewer component into your main App.js file.
import React from 'react';
import DicomViewer from './DicomViewer';
function App() {
const dicomUrl = 'wadouri:http://your-server.com/path-to-dicom-file.dcm';
return <DicomViewer dicomUrl={dicomUrl} />;
}
export default App;
Step 6: Test the Integration
npm start
-
Open the app in your browser.
-
Load a sample DICOM file.
- Test the zoom, pan, and image rendering capabilities.
Additional Features You Can Add
-
Zoom and Pan Tools: Allow users to zoom in and move around the image.
-
Measurement Tools: Enable measuring distances and angles within the image.
- Annotations: Let doctors mark critical areas on the image.
- Image Comparison: Show multiple images side by side.
-
Storage and Retrieval: Connect to a PACS system for better storage and image management.
Final Thoughts
Contact us for Custom Healthcare Solutions
Get in touch with us today to build a secure and scalable Hospital Management System!
Vaishali Gaudani
Skilled React.js Developer with 3+ years of experience in creating dynamic, scalable, and user-friendly web applications. Dedicated to delivering high-quality solutions through innovative thinking and technical expertise.
Reply